|TAYYABA MUNAWWAR
JavaScript stands as the cornerstone of modern web
development, powering a vast array of web applications
with its versatility and power. However, its complexity
can often lead developers into common pitfalls that
hinder functionality and lead to frustrating bugs. In
this article, we'll explore two prevalent issues in
JavaScript development and provide clear examples and
solutions to help you navigate these challenges
effectively.
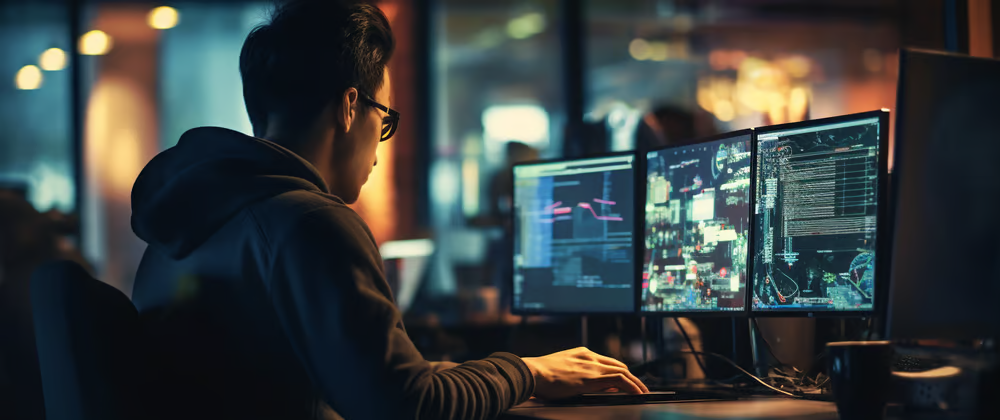
JavaScript Issue No. 1: Incorrect References to `this`
One of the most common pitfalls in JavaScript is the mishandling of the `this` keyword. Understanding how `this` behaves is crucial because its context is determined not by where a function is defined, but by how it is called. Let's look at an example:
// Incorrect use of `this`
var person = {
name: 'John',
greet: function() {
setTimeout(function() {
console.log('Hello, ' + this.name); // Incorrect:
`this` refers to the global object or undefined in
strict mode
}, 1000);
}
};
person.greet();
In this example, `this.name` inside the `setTimeout` callback does not refer to the `person` object as intended. Instead, it refers to the global object (`window` in browsers) or `undefined` in strict mode, because `setTimeout` runs the callback function in a different context.
To correctly reference `this`, you can use arrow functions or `Function.prototype.bind` to preserve the `this` context:
var person = {
name: 'John',
greet: function() {
setTimeout(() => {
console.log('Hello, ' + this.name); // Correct: `this`
refers to the `person` object
}, 1000);}
};
person.greet();
In this corrected version, an arrow function (`() => {}`) is used for the `setTimeout` callback, which inherits `this` from its surrounding lexical context (`greet` method), allowing `this.name` to correctly refer to the `person` object.
JavaScript Issue No. 2: Thinking There Is Block-level
Scope
Another common misconception involves variable scoping in JavaScript. Unlike languages like Java or C++, JavaScript's `var` keyword is function-scoped, not block-scoped. This can lead to unexpected behavior when variables are accessed outside their intended scope. Consider the following example:
// Incorrect understanding of block-level scope
function printNumbers() {
for (var i = 1; i = 5; i++) {
setTimeout(function() {
console.log(i); // Incorrect: `i` is function-scoped,
and all timeouts refer to the same variable `i`
}, i * 1000);
}}
printNumbers();
In this example, the `setTimeout` callbacks do not print the expected sequence of numbers (1, 2, 3, 4, 5). Instead, they all print `6` because the `i` variable is function-scoped due to `var`, and by the time each `setTimeout` callback executes, the loop has finished and `i` has the final value of `6`.
To achieve block-level scope, use `let` or `const`
instead of `var`:
function printNumbers() {
for (let i = 1; i = 5; i++) {
setTimeout(function() {
console.log(i); // Correct: `i` is block-scoped, and
each timeout callback refers to its own `i`
}, i * 1000);
}
}
printNumbers();
In this corrected version, `let i` is used in the `for` loop, creating a new variable `i` for each iteration. This ensures that each `setTimeout` callback correctly captures and prints the value of `i` at the time it was created, resulting in the expected sequence of numbers (1, 2, 3, 4, 5) printed with a one-second delay between each number.
Conclusion
By understanding and addressing these common JavaScript issues, you can write more robust and maintainable code. Mastery of JavaScript's nuances is essential for developing high-quality web applications. Stay tuned for more insights and best practices to enhance your JavaScript development skills!