| Dua Ali
Did you know regular expressions can revolutionize your
C# programming experience, allowing you to manipulate
and validate data efficiently? With C# regular
expressions, you can simplify complex pattern matching,
streamline
data validation, and supercharge your text manipulation tasks.
This article will delve into the world of regular
expressions in C#. We will explore their syntax, learn
how to apply them effectively and discover advanced
techniques to maximize their potential in
.NET projects.
If you're ready to unlock the power of C# regular
expressions, join us as we uncover the secrets of
efficient pattern matching, data validation, and text
manipulation in C#.
Key Takeaways:
- Regular expressions in C# simplify pattern matching, data validation, and text manipulation.
- Understanding the basic syntax and usage of regular expressions is crucial for effective implementation.
- Advanced techniques such as pattern replacement and specific pattern matching offer more flexibility.
- Regular expressions can handle various patterns, including email addresses and currency values.
- Regex options in C# provide additional control over the behavior of regular expressions.
Getting Started with C# Regex
Before diving into the details, let's start by
understanding the fundamentals of working with regular
expressions in C#. Regular expressions, or regex, are
powerful tools for pattern matching, data validation,
and text manipulation in .NET projects.
In C#, regular expressions are implemented using the
Regex class from the System.Text.RegularExpressions
namespace. This class provides various methods and
properties to work with regex patterns.
Using regular expressions in C# involves creating
patterns that define what you're searching for or
manipulating in a text. These patterns can range from
simple sequences of characters to more complex
combinations of characters, metacharacters, and
quantifiers.
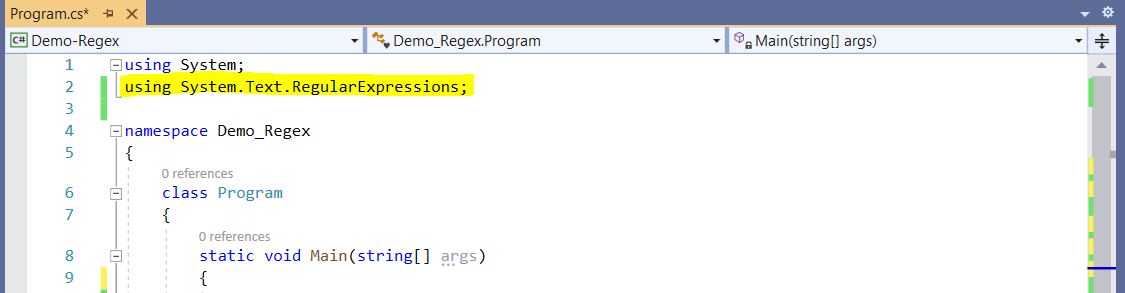
Regular expressions in C# can be used for various tasks, including
- Validating input data
- Extracting specific information from text
- Replacing text patterns
Let's look at how regular expressions work and how you can use them in your C# projects.
Regex Patterns and Metacharacters
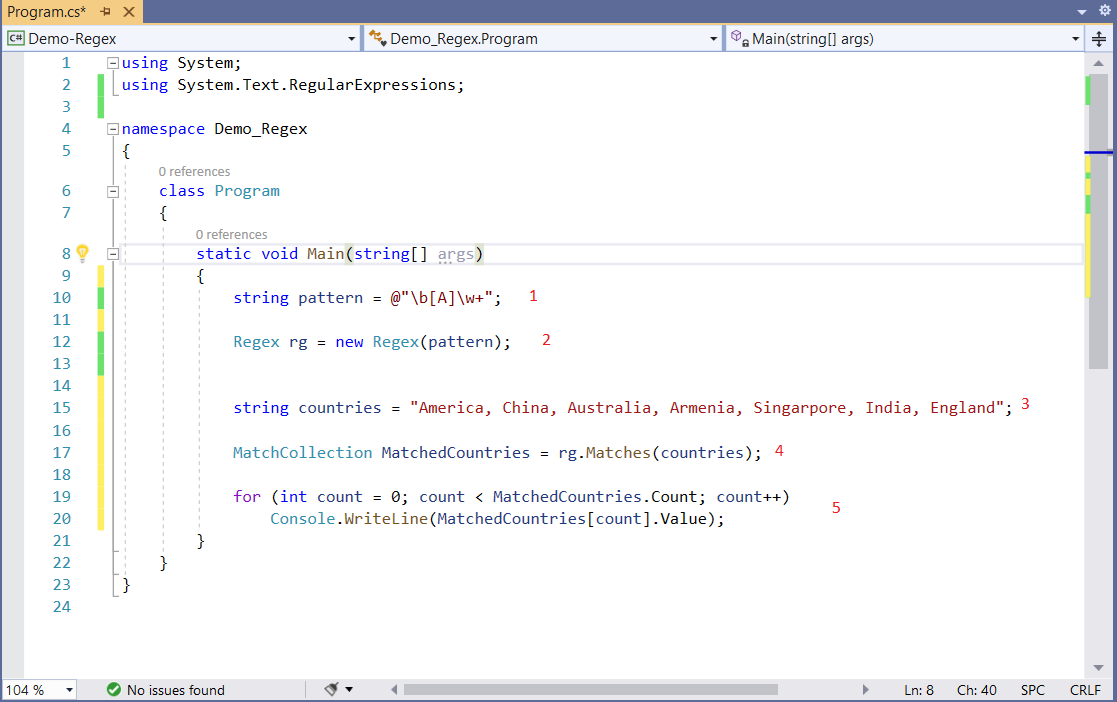
At the core of regular expressions in C# are patterns
and metacharacters. A pattern is a sequence of
characters that defines a search pattern. On the other
hand, Metacharacters have a special meaning in regular
expressions. They allow you to specify quantifiers,
character classes, anchors, etc.
Here are some commonly used metacharacters in C# regex:
Metacharacter | Description |
---|---|
. | Matches any single character |
\d | Matches any digit |
\w | Matches any word character (letter, digit, or underscore) |
\s | Matches any whitespace character |
[abc] | Matches any character within the brackets (a, b, or c) |
^ | Matches the start of a line |
$ | Matches the end of a line |
Combining these metacharacters with regular characters allows you to create powerful search patterns for matching or manipulating text.
Example: Matching Email Addresses
Consider a simple example of using regular expressions
in C# to match email addresses.
string pattern =
@"^[a-zA-Z0-9_.+-]+@[a-zA-Z0-9-]+\.[a-zA-Z0-9-.]+$";
string email = "example@example.com";
Match match = Regex.Match(email, pattern);
If (match. Success)
{
Console.WriteLine("Valid email address.");
}
else
{
Console.WriteLine("Invalid email address.");
}
In this example, we define a pattern that matches the
structure of a standard email address. We then use the
Regex.Match() method to test if the email address
matches the pattern. If it does, we consider it a valid
email address.
This is just a simple example, but it demonstrates the
power of regular expressions in C# and how they can be
used for data validation and pattern matching.
The following section will delve into the basic syntax
and usage of regular expressions in C#. We'll explore
how to create patterns and perform matching operations.
Basic Syntax and Usage
This section will explore the basic syntax and usage of regular expressions in C#. Regular expressions are powerful for matching patterns and text manipulation in .NET projects. Understanding the syntax and usage of regular expressions allows you to create patterns and perform matching operations.
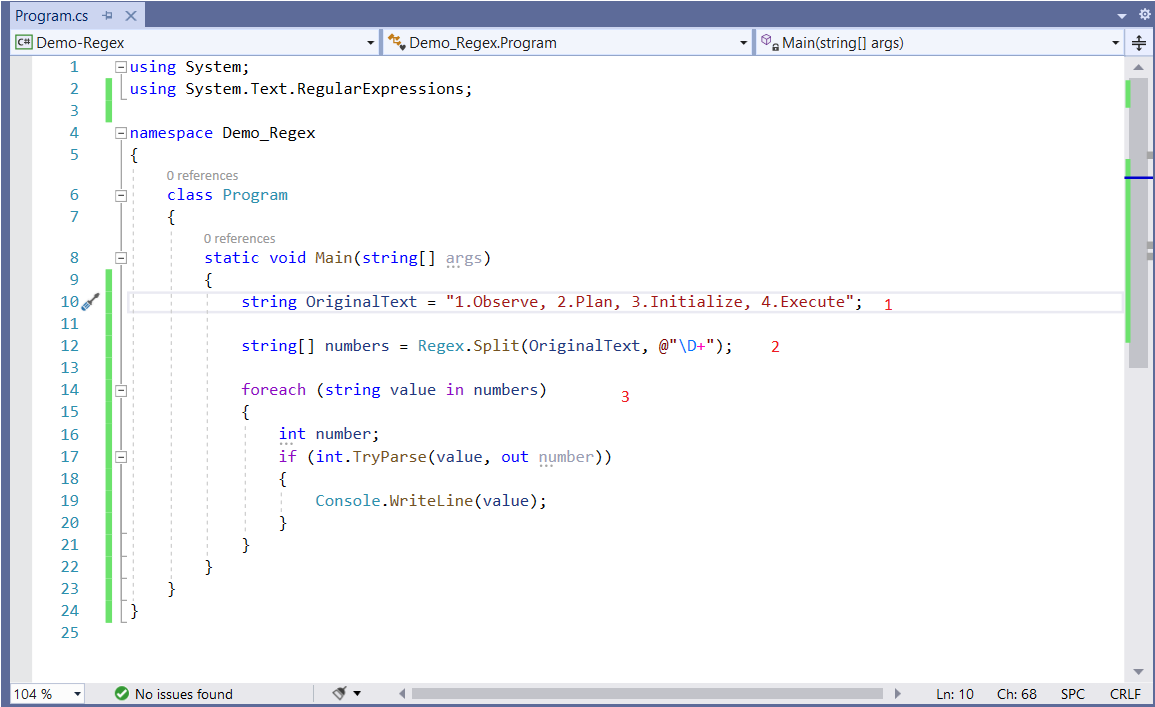
Create Patterns
When using regular expressions in C#, patterns are
created using a combination of characters and special
symbols. Here are some commonly used symbols:
. - Matches any character except a newline
* - Matches the preceding element zero or more times
+ - Matches the preceding element one or more times
? - Matches the preceding element zero or one time
[ ] - Matches any single character within the brackets
| - Matches the expression before or after the pipe
symbol.
These symbols can be combined and modified to create
complex patterns matching specific string patterns.
Perform Matching Operations
In C#, regular expressions are typically used with the
Regex. Match or Regex.Matches methods to perform
matching operations on strings.
Regex.Match - Returns the first occurrence of a pattern
in a string.
Regex. Matches - Returns all occurrences of a pattern in
a string.
These methods return a Match or MatchCollection object,
which can be used to obtain information about the
matched pattern and perform further operations.
Here's an example of using Regex.Match to find the first
occurrence of a pattern:
// Input string
string text = "The quick brown fox jumps over the lazy
dog";
// Pattern
string pattern = "brown";
// Perform matching operation
Match match = Regex.Match(text, pattern);
// Output the result
Console.WriteLine($"Match found: {match.Value}");
The above example will output:
Match found: brown
Similarly, Regex.Matches can be used to find all pattern
occurrences in a string.
Input | Pattern | Matches |
---|---|---|
The quick brown fox jumps over the lazy dog | o | o, o, o, o, o |
The rain in Spain falls mainly on the plain | ain | ain, ain, ain |
Advanced Regex Techniques
This section will explore more advanced techniques of using regular expressions in C#. Building on the knowledge gained in the previous section, we'll delve deeper into the power of regular expressions and discover how they can be used to replace patterns, match specific patterns, and more.
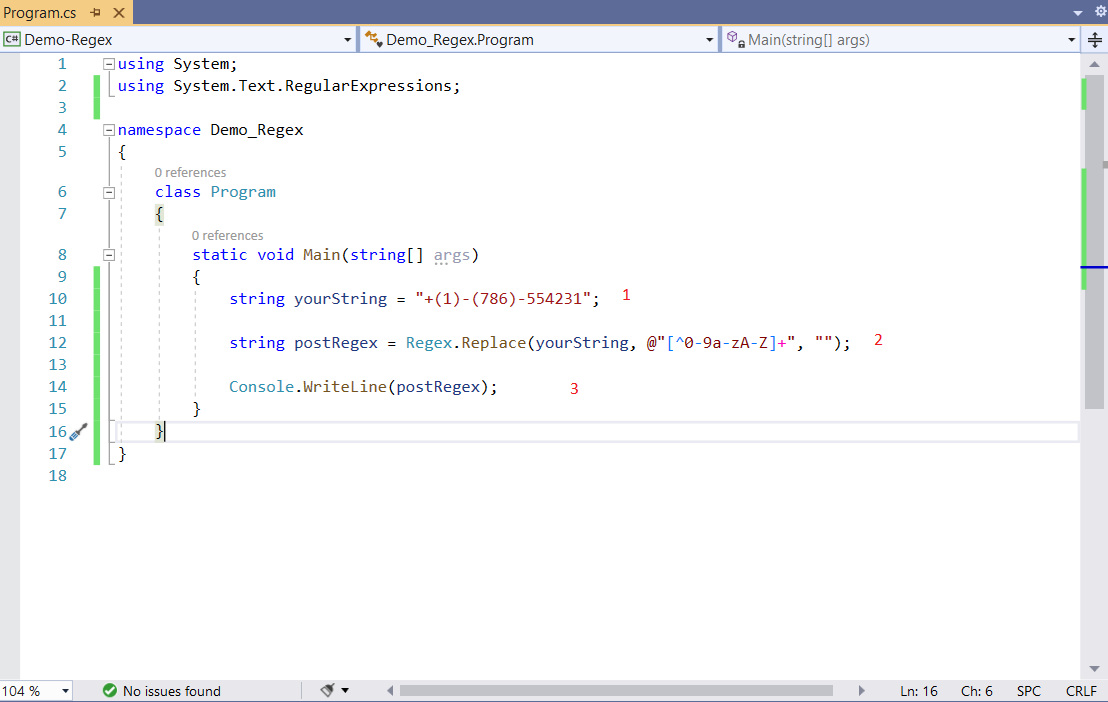
Replacing Patterns with C# Regex
One of the most powerful features of regular expressions in C# is the ability to replace patterns within a given text. Using the c# regex replace method, you can effortlessly search for a specific pattern and replace it with desired content. This allows for efficient text manipulation and data processing, saving time and effort in your coding endeavors.
Matching Specific Patterns with C# Regex
Another critical aspect of working with regular
expressions in C# is matching specific patterns. With
the c# match pattern capability, you can specify a regex
pattern to search for within a given text and retrieve
the matching results. This enables you to extract
specific information from a string, validate input, or
perform complex pattern-matching tasks.
By utilizing the regex replace pattern c# and c# match
pattern, you can unlock many possibilities in your C#
development projects. Whether you need to manipulate
text, validate data, or perform complex pattern
matching, regular expressions in C# provide the tools
you need to achieve your goals.
Regular expressions are a game-changer in C#
development. With the ability to replace patterns and
match specific patterns, you can simplify your coding
tasks and achieve more efficient and elegant solutions.
Stay tuned for the next section, where we'll explore
various examples of patterns that can be used with
regular expressions in C#. Through practical examples,
you'll gain a deeper understanding of how to leverage
the power of regex in your projects.
Regex Technique | Description |
---|---|
Replacing Patterns | Allows for efficient text manipulation by replacing specific patterns within a given text |
Matching Specific Patterns | Enables the extraction of specific information or validation of input by matching predefined patterns. |
Working with Different Patterns
Regular expressions in C# offer a powerful way to work with various patterns, allowing you to perform advanced text manipulation, matching, and validation operations. This section will explore practical examples of patterns you can use with regular expressions in C#.
Matching Email Addresses
Email addresses follow a specific pattern, and regular
expressions can help you efficiently validate and
extract email addresses from text. Here's an example of
a regular expression pattern that matches email
addresses:
[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}
Using this pattern, you can quickly validate whether a
given string follows the standard email address format
in C#.
Matching Currency Values
Regular expressions can simplify validating and
extracting these values from text if you need to work
with currency values in your C# application. Here's an
example of a regular expression pattern that matches
currency values:
\$[0-9]{1,3}(?:,?[0-9]{3})?(?:\.[0-9]{2})?
This pattern allows you to match currency values
in dollars and cents, with optional thousands of
separators and decimal points.
Replacing Email Addresses
Regular expressions in C# can also replace specific
patterns within a string. For example, if you want to
hide or replace email addresses in a text, you can use
the following regular expression pattern:
(?i)\b[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,}\b
Using this pattern and the Regex.Replace method in C#.
You can easily replace email addresses with a masked or
dummy value.
Pattern | Description |
---|---|
[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,} | Matches email addresses. |
\$[0-9]{1,3}(?:,?[0-9]{3})?(?:\.[0-9]{2})? | Matches currency values. |
(?i)\b[A-Z0-9._%+-]+@[A-Z0-9.-]+\.[A-Z]{2,}\b | Matches email addresses for replacement. |
These examples demonstrate a few possibilities when using regular expressions in C# with different patterns. By leveraging the power of C# regex, you can handle complex data validation and manipulation tasks more efficiently.
Handling Date Formatting
Date formatting is a common task in many applications.
Whether capturing user input, working with APIs, or
storing data in databases, handling different date
formats is crucial. Regular expressions can be a
powerful tool in C# to ensure consistent and precise
date formatting.
Regular expressions provide a flexible and efficient way
to match and manipulate text patterns. You can create
dynamic patterns that match specific date formats and
extract relevant information from date strings by
leveraging regular expressions. This allows you to
validate, parse, and transform data according to your
application's requirements.
Why Use Regular Expressions for Date Formatting?
Using regular expressions for date formatting in C# offers several advantages:
- Flexibility: Regular expressions allow you to define custom date formats and handle a wide range of date representations, such as "MM/dd/yyyy," "dd-MM-yyyy," or "yyyy/MM/dd."
- Pattern Matching: With regular expressions, you can easily identify and validate if a given date string matches the expected format, ensuring data integrity.
- String Manipulation: Regular expressions enable you to extract and transform specific date components, such as the day, month, or year from a date string.
Common Regular Expressions for Date Formatting
Here are some common regular expressions that can be
used for date formatting in C#:
"\d{2}/\d{2}/\d{4}" - Matches dates in the format
month/day/year, such as "01/24/2022".
"\d{4}-\d{2}-\d{2}" - Matches dates in the format
year-month-day, such as "2022-01-24".
"\d{2}-\d{2}-\d{4}" - Matches dates in the format
day-month-year, such as "24-01-2022".
These regular expressions can be a starting point for
handling common date formats. However, it's essential to
customize them according to the specific date formats
you encounter in your application.
Implementing Date Formatting with Regular Expressions
Implementing date formatting with regular expressions in C# involves several steps:
- Construct a regular expression pattern that matches the desired date format.
- Use the Regex.Match or Regex.IsMatch methods to check if a date string matches the pattern.
- If a match is found, extract the relevant date components using capturing groups or other regex techniques.
- Perform necessary transformations, such as converting the extracted components to a standardized date format or performing calculations.
Following these steps, you can handle various date formats effectively and ensure consistent date processing in your C# applications.
Regex Options in C#
C# offers several options and flags that can be used with regular expressions. These options allow you to modify the behavior of regular expressions and customize them to suit your specific needs. Whether you're parsing complex data or searching for patterns in a text, these regex options can help optimize your code and improve performance.
Parse Regular Expression
One essential option in C# regex is the ability to parse
a regular expression and convert it into a Regex object.
This is done using the RegexOptions.Compiled option
compiles the regular expression pattern into a more
efficient format for faster matching.
Here's an example of how you can use the
RegexOptions.Compiled option:
string pattern = "your regex pattern";
Regex regex = new Regex(pattern, RegexOptions.Compiled);
Other Regex Options
In addition to the RegexOptions.Compiled option, C# provides several other useful options:
- RegexOptions.IgnoreCase: This option enables case-insensitive matching, allowing you to ignore the difference between uppercase and lowercase letters.
- RegexOptions.Multiline: When this option is used, the ^ and $ anchors match each line's beginning and end rather than the entire string's beginning and end.
- RegexOptions.Singleline: This option changes the behavior of the dot (.) metacharacter to match any character, including newline characters.
- RegexOptions.ExplicitCapture: By default, capturing groups in a regex pattern are assigned numbers. This option allows you to name-capture groups and refer to them by name instead of number.
These options can be combined using the bitwise OR
operator (|) to create customized regex behavior that
meets your requirements.
Understanding and utilizing these regex options in C#
can greatly enhance your ability to handle complex
pattern-matching and data manipulation tasks. By
leveraging the power of regular expressions alongside
these options, you can optimize your code and improve
the efficiency of your applications.
Validating and Manipulating Text
Regular expressions are powerful tools for validating
and manipulating text in C#. With their versatile
pattern-matching capabilities, regular expressions
provide a flexible solution for tasks such as data
validation, input sanitization, and text extraction.
Let's examine how regular expressions can validate and
manipulate text in different scenarios.
Validating Email Addresses
Email addresses are commonly used in web applications
for user registration and communication. Validating
email addresses ensures the input follows a specific
format and avoids accepting invalid or malformed
addresses.
In C#, you can use regular expressions to validate email
addresses by checking for specific patterns.
\b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}\b
The above regular expression checks for the basic
structure of an email address. It verifies that the
address contains alphanumeric characters, special
characters like dot (.), underscore (_), percent (%),
and (+) before the "@", and a valid domain after the
"@".
Validating Currency Format
Validating currency formats is crucial in financial
applications and e-commerce platforms to ensure that the
input follows the correct monetary format. Regular
expressions can be used to validate and extract currency
values from text.
Here's an example of a regular expression that validates
currency in a specific format:
\$[0-9]{1,3}(?:,?[0-9]{3})*(?:\.[0-9]{2})?
This regular expression validates currency values with
thousands of optional separators (commas) and ensures
the value has up to two decimal places.
Replacing Text Patterns
Regular expressions are also handy for replacing
specific patterns in text. In C#, you can use regular
expressions for find and replace operations, enabling
you to manipulate text effectively.
For example, you can use a regular expression to replace
all occurrences of a specific word or phrase in a
string:
Regex.Replace(inputText, "oldWord", "newWord");
This will replace all occurrences of "oldWord" with
"newWord" in the input text.
Manipulating Text with Capturing Groups
Regular expressions support capturing groups, which
allow you to extract specific parts of a matched
pattern. This feature can be useful when you need to
extract and manipulate specific portions of a text.
For example, you can use a capturing group to extract
the username from an email address:
Regex.Match(email,
@"^([a-zA-Z0-9_\-\.]+)@([a-zA-Z0-9_\-\.]+)\.([a-zA-Z]{2,5})$").Groups[1].Value;
This extracts the username part of the email address
before the "@" symbol.
Regular expressions provide a powerful and flexible way
to validate and manipulate text in C#. By understanding
the syntax and using the appropriate patterns, you can
efficiently handle a wide range of text-related tasks in
your .NET projects.
Use Cases | Regular Expression |
---|---|
Validating Email Addresses | \b[A-Za-z0-9._%+-]+@[A-Za-z0-9.-]+\.[A-Za-z]{2,}\b |
Validating Currency Format | \$[0-9]{1,3}(?:,?[0-9]{3})*(?:\.[0-9]{2})? |
Replacing Text Patterns | Example: Regex.Replace(inputText, "oldWord", "newWord"); |
Manipulating Text with Capturing Groups | Example: Regex.Match(email, @"^([a-zA-Z0-9_\-\.]+)@([a-zA-Z0-9_\-\.]+)\.([a-zA-Z]{2,5})$").Groups[1].Value; |
Tutorial: Building a Regex-Driven Application
In this tutorial section, we'll walk through the process of building a Regex-driven application in C#. Regular expressions, also known as regex, are powerful tools for pattern matching and text manipulation in programming. By understanding how to use regex effectively, you can enhance the functionality and flexibility of your C# applications.
Why Use Regular Expressions in Your Application?
Regular expressions provide a concise and efficient way to search for specific patterns within text and perform various operations, such as validating user input or extracting specific data. By leveraging regex patterns in your application, you can simplify complex string manipulation tasks and achieve more accurate and efficient results.
Best Practices for Using C# Regex Patterns
When working with regular expressions in C#, it's important to follow best practices to ensure the reliability and maintainability of your code. Here are some tips:
- Start with a clear objective: Identify the specific pattern you want to match or manipulate.
- Test and validate your regex pattern: Use online regex testers or built-in regex tools in your development environment to verify the correctness of your pattern.
- Consider performance implications: Regular expressions can be resource-intensive, so optimize your patterns to achieve better performance when working with large datasets.
- Document your regex patterns: Provide clear explanations and comments to make it easier for other developers (and yourself) to understand and modify the patterns in the future.
- Use regex options and modifiers when necessary: C# provides various options and flags that you can apply to modify the behavior of regular expressions, such as case sensitivity or multiline matching.
Walkthrough: Building a Regex-Driven Application
In this walkthrough, we'll demonstrate the process of building a C# console application that uses regex patterns to validate and manipulate user input. We'll create a simple form validation scenario where the user is prompted to enter an email address and a password.
- Create a new C# console application project in your preferred development environment.
- Add the necessary input validation logic using regular expressions to ensure the email address and password meet the required criteria.
- Test the application by entering various email addresses and passwords to ensure the validation works correctly.
- Enhance the application by adding additional regex patterns to validate other input fields, such as phone numbers or postal codes.
- Document your code and add comments to explain the purpose and functionality of the regex patterns you've used.
By following this tutorial and applying the principles of regex, you'll have a solid foundation for building regex-driven applications in C#. With regular expressions, you can unlock the power of pattern matching and text manipulation, making your applications more versatile and efficient.
Conclusion
In conclusion, regular expressions in C# offer
developers a robust and versatile tool for handling
pattern matching, data validation, and text manipulation
in .NET projects. With a thorough understanding of C#
regex, developers can simplify complex tasks and
streamline development.
Regular expressions provide a concise and powerful
syntax for defining patterns, making searching and
extracting specific information from strings easier.
Whether you need to validate user input, extract data
from a text file, or replace specific patterns in a
document, C# regex can help you achieve these goals
efficiently.
By leveraging the power of regular expressions,
developers can enhance the functionality of their
applications and improve the overall user experience.
Regular expressions can save development time and effort
with proper implementation, enabling developers to focus
on other critical aspects of their projects.
Stay tuned for more articles on C# and regex, where we
will delve deeper into specific use cases, advanced
techniques, and practical examples. Mastering regular
expressions in C# will undoubtedly open up new
possibilities and empower you to tackle even the most
complex challenges in your coding journey!
FAQ
What are regular expressions?
Regular expressions, or regex, are powerful tools for pattern matching and manipulating text in C# and other programming languages. They provide a concise and flexible way to search, replace, and validate strings based on complex patterns.
How can regular expressions be used in C#?
Regular expressions can be used in C# to perform tasks such as validating user input, extracting specific data from strings, manipulating and transforming text, and more. They are commonly used in form validation, data parsing, and text processing.
How do I create a regex pattern in C#?
In C#, you can create a regex pattern using the `Regex` class provided by the .NET Framework. You can construct a regex pattern using symbols and modifiers to define the desired matching criteria.
What are some everyday use cases for regular expressions in C#?
Regular expressions can be used in C# for various tasks, including: - Validating email addresses or other input formats. - Searching and extracting specific patterns from text. - Replacing specific patterns in a string with another value. - Parsing and validating dates, times, and other formats. - Identifying and extracting numbers, URLs, and other specific data types. These are just a few examples, and the possibilities for using regular expressions in C# are virtually endless.
Are regular expressions case-sensitive in C#?
By default, regular expressions in C# are case-sensitive. This means that the regex pattern will match strings with the same casing specified in the pattern. However, you can use regex options such as `RegexOptions.IgnoreCase` to make the matching process case-insensitive.
Can I use regular expressions to validate an email address in C#?
Yes, regular expressions commonly validate email addresses in C#. You can create a regex pattern that matches the required email format, including the correct placement of the "@" symbol, domain name, and top-level domain.
Are there online resources or tools for testing regular expressions in C#?
Yes, various online resources and tools are available for testing and debugging regular expressions in C#. Some popular options include regex101.com, regexr.com, and RegexBuddy. These tools provide a convenient way to validate and experiment with regex patterns.