| IMPERIUM DYNAMICS
Data types in Power Automate are crucial for ensuring your workflows function correctly. Integer (Int) and Decimal are two common types used for numbers. Using the wrong one can lead to errors or even stop your flow.
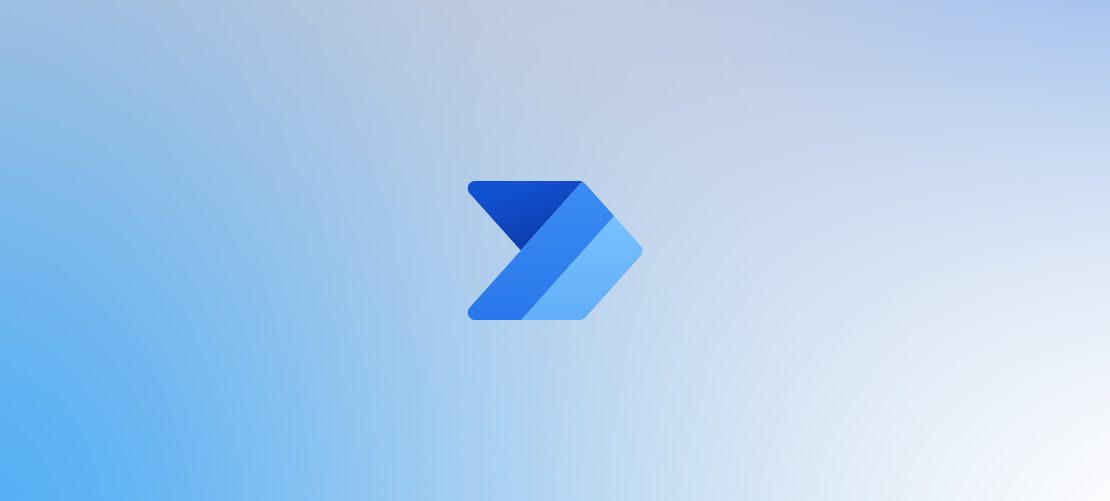
In this article, we'll explore the differences between Int and Decimal in Power Automate. We'll discuss when to use each and share tips to help you avoid common mistakes.
Understanding Integer (Int) in Power Automate
What is an Integer?
An Integer, or Int, is a whole number. It has no fractions or decimal points. Examples include -10, 0, or 25.
When to Use Int
- Loop counters or indexes
- Counting items
-
Conditional logic where only whole numbers are
valid
Characteristics:
-
Lightweight and efficient in memory usage
- Fast to process
-
Unsuitable for financial or fractional values
Example:
Using an Int in a loop that sends an email 5 times:
CODE:
Initialize variable:
Name: Counter
Type: Integer
Value: 1
Understanding Decimal in Power Automate
What is a Decimal?
A Decimal represents numbers with fractional parts. Examples include 3.14, -0.75, or 99.99.
When to Use Decimal
- Financial or currency calculations
- Percentages or averages
- Measurement data that includes fractions
Characteristics:
- Supports precision
- Ideal for calculations requiring accuracy
- Slightly heavier in terms of memory
Example:
Calculating a 10% discount on a product price:
CODE:
Price = 100
Discount = Price * 0.10 // Returns 10.0 as Decimal
Key Differences Between Int and Decimal
Feature | Integer (Int) | Decimal |
---|---|---|
Definition | Whole numbers | Numbers with fractional parts |
Precision | No decimal support | High precision |
Memory Usage | Lower | Higher |
Use Cases | Looping, counters | Financial calculations, percentages |
Performance | Faster | Slightly slower |
Conversion | Requires explicit conversion | Requires explicit conversion |
Data Type Behavior in Expressions
Integer (Int)
Definition: Represents whole numbers without any fractional component (e.g., -10, 0, 25).
Behavior: When performing arithmetic operations, if all operands are integers, the result will also be an integer. This means that any fractional part will be truncated.
Code Example:
div(5, 2) // Returns 2, not 2.5
In this example, dividing two integers results in an
integer output, truncating the decimal part.
Decimal
Definition: Represents numbers with fractional components, offering higher precision (e.g., 3.14, -0.75, 99.99).
Behavior: When at least one operand in an arithmetic operation is a decimal, the result will be a decimal, preserving the fractional component.
Code Example:
div(5.0, 2) // Returns 2.5
Here, using a decimal operand ensures that the division
retains the fractional part.
Data Type Conversion and Compatibility
Power Automate provides functions to convert between data types, ensuring compatibility in operations:
Int () Function: Converts a value to an integer. Useful when you need to ensure a number is a whole number.
Code Example:
int('123.45') // Returns 123
Float () Function:
Converts a value to a floating-point number, which can
represent decimal values.
Code Example:
float('123.45') // Returns 123.45
Decimal () Function: Specifically
converts a value to a decimal number, ensuring precision
in calculations.
Code Example:
decimal('123.45') // Returns 123.45
These functions are beneficial when dealing with data
inputs that may not be in the expected format, such as
strings representing numbers.
Formatting Numbers for Output
When presenting numbers in outputs like emails or
reports, formatting enhances readability:
formatNumber() Function: Formats a
number according to specified format strings and
locales.
Code Example:
formatNumber(12345.6789, 'C2', 'en-US') // Returns
$12,345.68
This function is beneficial for displaying currency,
percentages, or numbers with a specific number of
decimal places.
Common Pitfalls and How to Avoid Them
1. Type Mismatch Errors
Mixing Int and Decimal in calculations or conditions can
lead to unexpected results or failed flows.
Solution:
Use conversion functions like float() or int() when
needed.
2. Unexpected Rounding
Using an Int in place of Decimal may cause values to be
rounded off prematurely.
Solution:
Always use Decimal when precision matters, especially
with currency.
3. Formatting Issues
Decimals might show too many digits or round awkwardly.
Solution:
Use Power Automate expressions or string formatting to
resolve this issue.
Best Practices
- Be Consistent: Always use the same type in calculations to avoid problems.
- Convert Explicitly: Utilize built-in functions to convert values explicitly, rather than relying on automatic conversion.
- Validate Inputs: Use expressions to check if a number is an integer or float before processing.
- Use Descriptive Names: Name your variables clearly to indicate their type (e.g., totalPriceDecimal, itemCountInt).
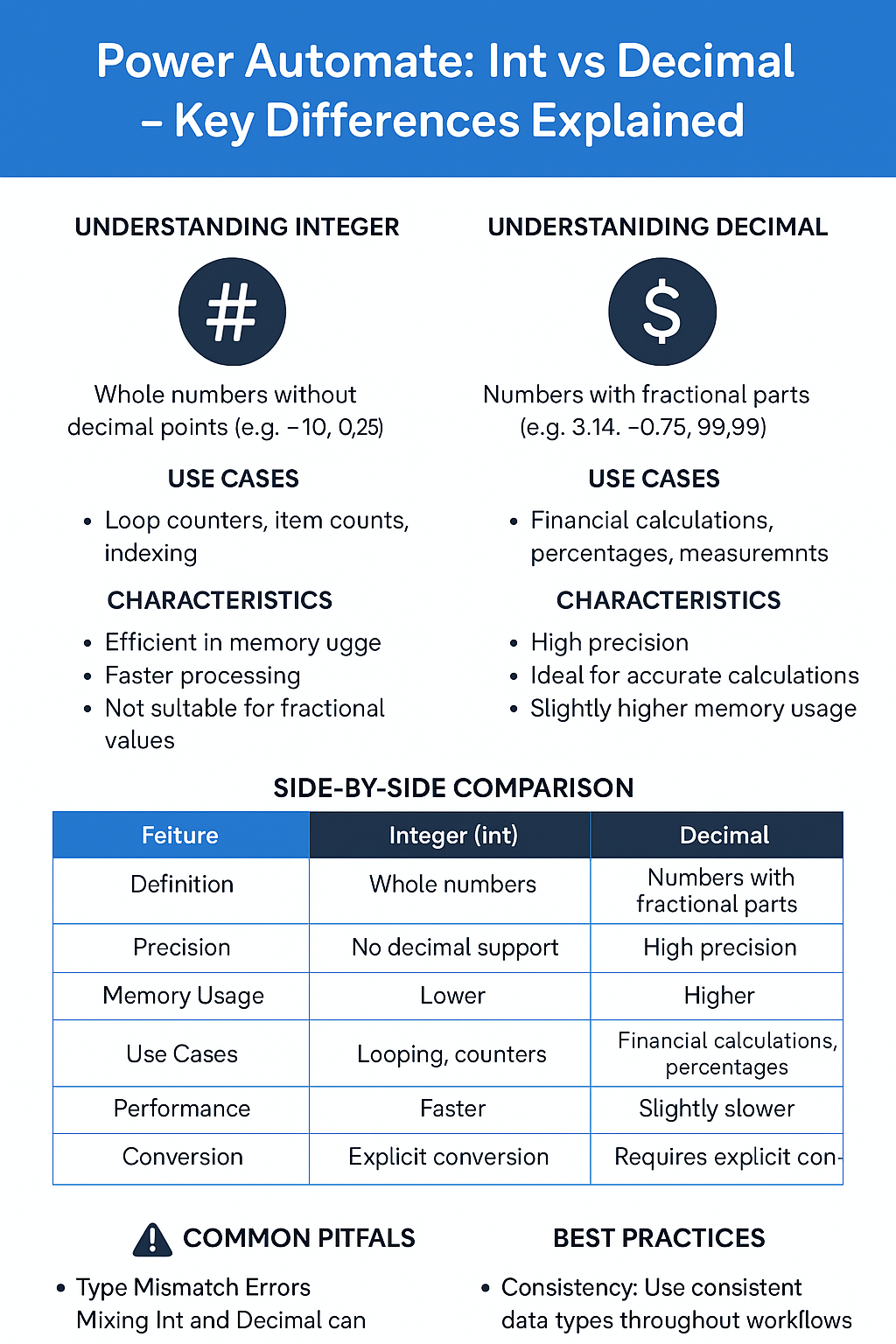
Conclusion
Choosing between
Int
and
Decimal
in Power Automate is crucial. It affects how your
workflows work, especially with logic, iterations, or
calculations. Understanding the
key differences
and selecting the appropriate data type is crucial for
creating more effective workflows.
Whether you're making a simple counter or handling
complex financial tasks, remember these tips. They help
ensure your automations work effectively.