| Tayyaba Munawwar
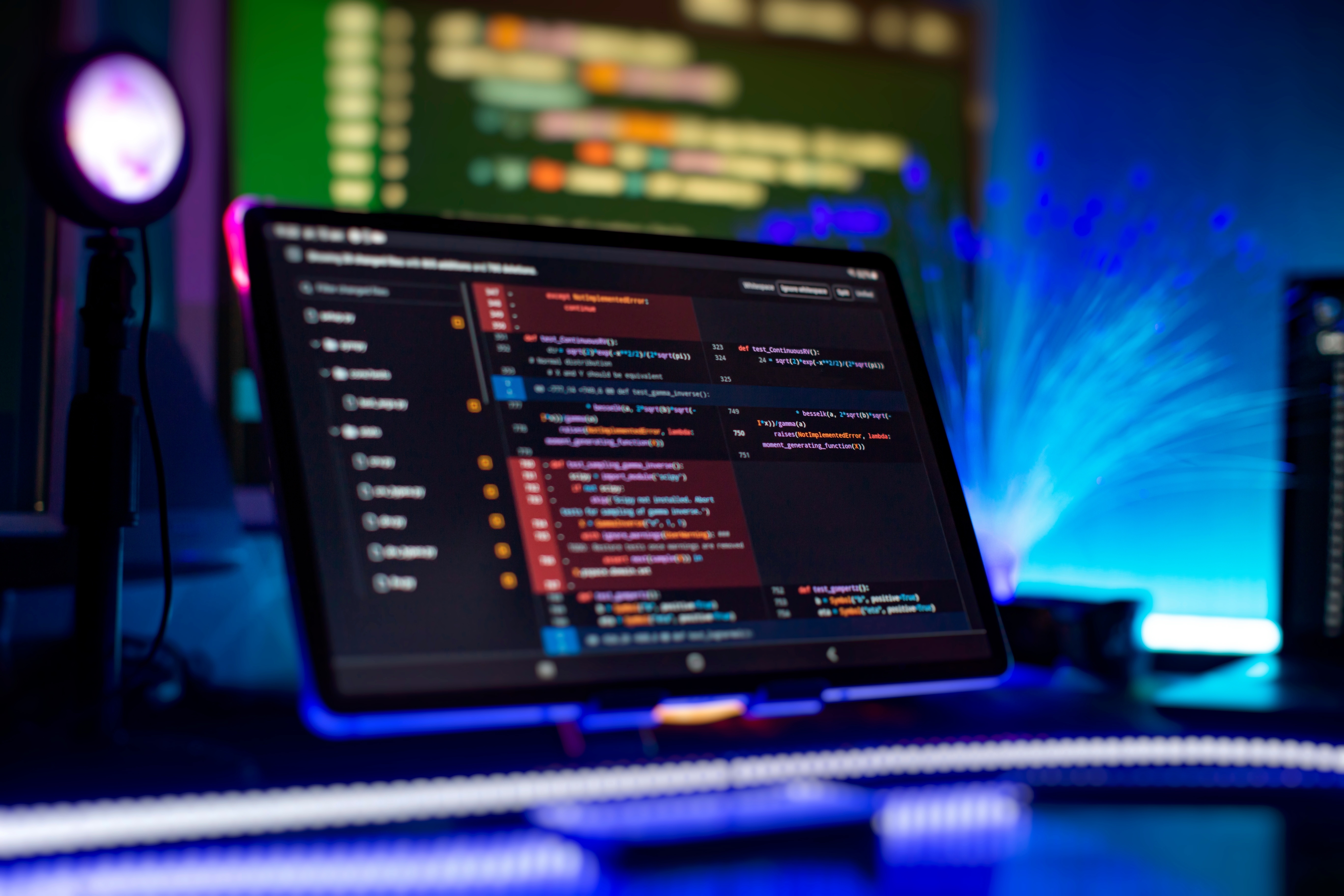
Asynchronous programming in JavaScript is vital for acting tasks like fetching records from APIs, interacting with databases, or studying files, all without blocking the principle thread. Over time, the approach to dealing with asynchronous tasks has developed from simple callbacks to more sophisticated solutions like Promises and `async/wait for`. Let’s explore how each of these patterns works and why `async/watch for` is taken into consideration the contemporary trendy.
1. Callbacks
Callbacks have been the authentic manner to handle asynchronous code in JavaScript. A callback is a characteristic handed as an argument to some other function, performed as soon as the venture completes. While purposeful, callbacks can effortlessly cause "callback hell" whilst a couple of nested operations are concerned, making the code tough to examine and hold.
function fetchData(callback) {
setTimeout(() => {
callback("Data loaded");
}, 1000);
}
fetchData(function(result) {
console.log(result);
});
In this example, the callback executes after a delay, and the code structure can get messy if there are multiple tasks to chain together.
2. Promises
Promises provide a greater dependent and cleaner method to handle asynchronous operations. A promise represents the eventual of completion (or failure) of an asynchronous operation and its ensuing price. With `.Then()` for success and `.Capture()` for mistakes, Promises keep away from the nesting problems that arise with callbacks.
// Promise example
function fetchData() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve("Data loaded");
}, 1000);
});
}
fetchData().then(result => {
console.log(result);
}).catch(error => {
console.error(error);
});
Promises are cleaner, but chaining multiple async operations with `.then()` can sometimes still become hard to read when dealing with more complex scenarios.
3. Async/Await
`async/await` is the most recent and preferred way to handle asynchronous code in JavaScript. Introduced in ECMAScript 2017, `async/await` allows asynchronous code to be written in a more synchronous manner, improving readability and reducing complexity. The `async` keyword is used to define an asynchronous function, and `await` pauses the function execution until the Promise resolves, making the code look much cleaner and easier to follow.
// Async/Await example
async function fetchData() {
return new Promise(resolve => {
setTimeout(() => {
resolve("Data loaded");
}, 1000);
});
}
async function loadData() {
const result = await fetchData();
console.log(result);
}
loadData();
In this example, `await` makes the asynchronous `fetchData()` function behave like a synchronous function, meaning the result is available immediately after `await` without needing `.then()`.
Why `async/await` is the Best Approach
-
Readability:`async/await` makes asynchronous code look and behave more like synchronous code, which is easier to read and debug.
-
Error Handling: With `try/catch` blocks, error handling becomes more straightforward, as opposed to chaining `.catch()` with Promises.
-
Maintainability:The simpler syntax of `async/await` helps keep codebases cleaner, especially when there are complex sequences of asynchronous operations.
// Handling errors with async/await
async function loadData() {
try {
const result = await fetchData();
console.log(result);
} catch (error) {
console.error("An error occurred:", error);
}
}
loadData();
// Handling errors with async/await
async function loadData() {
try {
const result = await fetchData();
console.log(result);
} catch (error) {
console.error("An error occurred:", error);
}
}
loadData();
In conclusion, JavaScript’s evolution from callbacks to Promises and finally to `async/await` has made it significantly easier to work with asynchronous tasks. `async/await` is now widely adopted due to its simplicity and powerful error handling, making it the go-to solution for modern JavaScript developers.
FAQs
1. What is the difference between a callback and a promise?
A callback is a function passed to another function to be executed after the completion of an operation. However, callbacks can result in nested code structures that are hard to manage.
A promise is an object that represents the eventual completion (or failure) of an asynchronous operation. Promises allow chaining operations, which makes asynchronous code more readable and manageable.
2. Can I use `async/await` with callbacks?
Yes, you can use `async/await` with callbacks by wrapping the callback-based function inside a Promise. This allows you to take advantage of the cleaner syntax that `async/await` provides.
3. How do I handle errors with `async/await`?
Error handling with `async/await` is straightforward using `try/catch` blocks. If an error occurs within an `await` expression, it is automatically caught by the `catch` block.
try {
const data = await fetchData();
console.log(data);
} catch (error) {
console.error("Error occurred:", error);
}
4. Is `async/await` always better than Promises?
`async/await` is often preferred for its simplicity and readability, especially when dealing with multiple asynchronous operations. However, Promises are still useful, particularly when chaining multiple `.then()` operations or handling multiple concurrent operations.
5. Can `async/await` be used with existing promise-based APIs?
Yes, `async/await` works seamlessly with promise-based APIs. Any function that returns a promise can be used with `await` to pause execution until the promise resolves.