| Tayyaba Munawwar
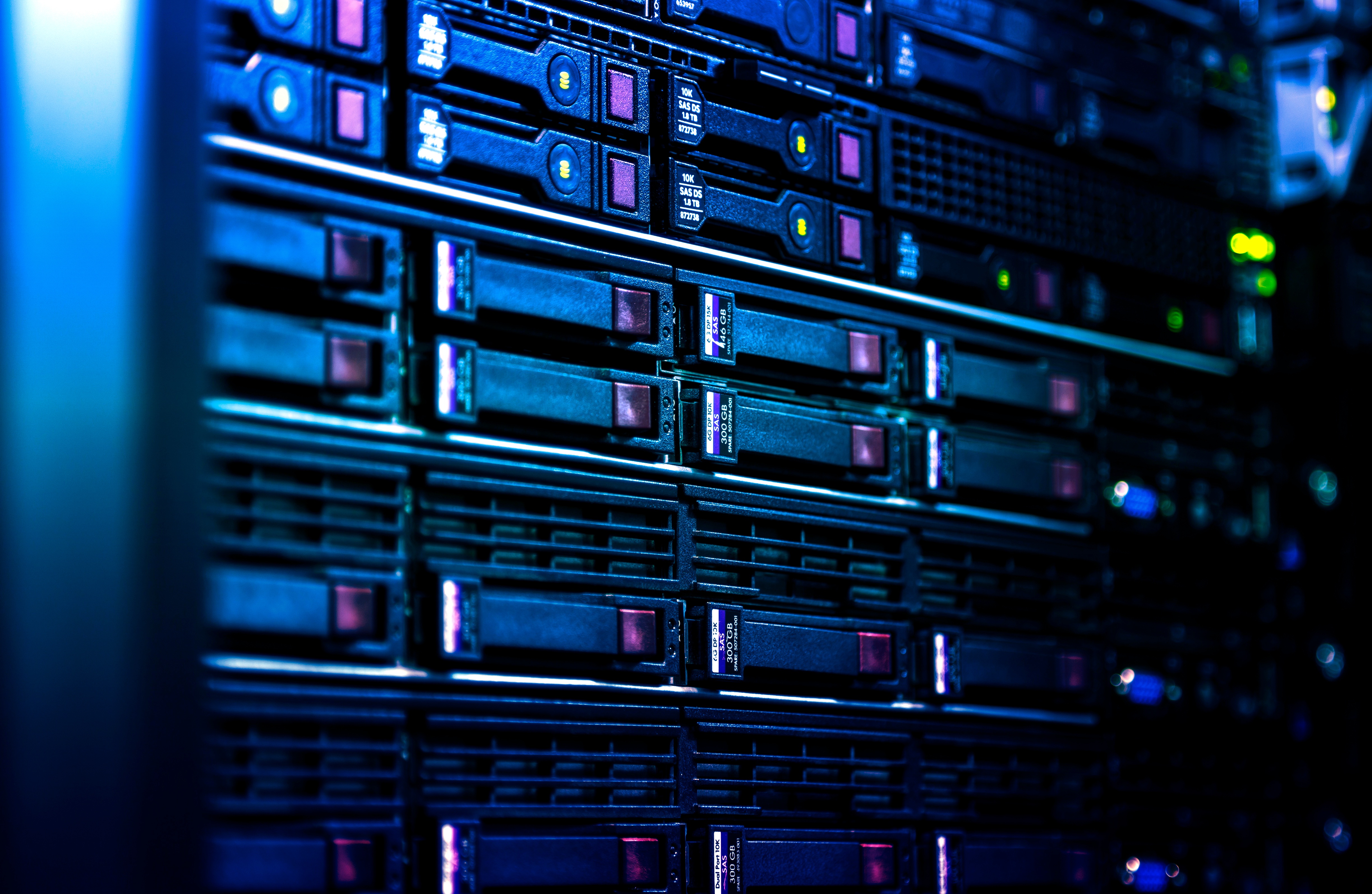
In cutting-edge web development, handling customer-aspect statistics is a crucial aspect of delivering fast, responsive, and seamless person stories. The browser offers a whole lot of storage alternatives for builders to persist facts on the patron side, of the maximum popular being Local Storage and IndexedDB . Both are useful, however each has its strengths and weaknesses. In this weblog, we will discover each technologies, comparing them and imparting pointers on while to use each for different eventualities.
This article explains using the Dynamics 365 cost calculator and price list. It will help companies make smart choices that fit their budgets and goals.
What is Local Storage?
Local Storage is a part of the Web Storage API and allows developers to save key-value pairs in the browser’s nearby garage. It’s widely used for simple facts storage needs like saving consumer possibilities, session data, or other small quantities of data.
- Capacity: Typically limited to around 5MB per domain.
- Synchronous: Local Storage operations are synchronous, meaning that data is stored or retrieved immediately, which can block the UI thread if there are large amounts of data.
- Simple API: It has a very easy-to-use API for storing and retrieving data.
// Setting an item in Local Storage
localStorage.setItem('username', 'john_doe');
// Getting an item from Local Storage
let username = localStorage.getItem('username');
console.log(username); // Output: john_doe
// Removing an item
localStorage.removeItem('username');
Use Case for Local Storage
Local Storage is best for storing small statistics like user possibilities, subject matters, or simple authentication tokens. It's clean to apply and provides a straightforward solution for non-touchy data that does not need to be complicated or hierarchical.
What is IndexedDB?
IndexedDB, alternatively, is a low-stage, asynchronous API designed for storing large amounts of dependent statistics. Unlike Local Storage, it permits you to store more complex data kinds, which includes gadgets, arrays, and binary statistics. IndexedDB is often used for scenarios wherein more advanced features are wanted, like storing offline information, caching huge datasets, or building purchaser-facet databases for complex packages.
- Capacity: Much larger than Local Storage, often in the hundreds of MBs or more, depending on the browser and device.
- Asynchronous: IndexedDB operations are asynchronous, meaning they won’t block the UI thread, ensuring a smoother user experience.
- Structured Storage: Allows storing rich, structured data, such as objects, and supports indexing, which makes querying more efficient.
// Opening or creating an IndexedDB database
let request = indexedDB.open('myDatabase', 1);
// Creating object stores and indexes when upgrading the database version
request.onupgradeneeded = function(event) {
let db = event.target.result;
let store = db.createObjectStore('users', { keyPath: 'id' });
store.createIndex('name', 'name', { unique: false });
};
// Storing data in IndexedDB
request.onsuccess = function(event) {
let db = event.target.result;
let transaction = db.transaction(['users'], 'readwrite');
let store = transaction.objectStore('users');
store.add({ id: 1, name: 'John Doe', email: 'john@example.com' });
};
// Retrieving data from IndexedDB
function getUserData(id) {
let transaction = db.transaction(['users'], 'readonly');
let store = transaction.objectStore('users');
let request = store.get(id);
request.onsuccess = function() {
console.log(request.result); // Output: {id: 1, name: 'John Doe', email: 'john@example.com'}
};
}
Use Case for IndexedDB
IndexedDB is ideal for more complex applications where performance and data structure are important. It’s useful for scenarios like building Progressive Web Apps (PWAs) that need to work offline, or for applications that store large datasets like product catalogs, user data, or even media files. IndexedDB is also the go-to solution for storing data for applications that need rich queries, indexing, and fast lookups.
Local Storage vs. IndexedDB: A Comparison
Feature | Local Storage | Local Storage |
---|---|---|
Data Type | Simple key-value pairs | Structured data (objects, arrays, etc.) |
Capacity | ~5MB per domain | Much larger (100MB or more) |
Synchronous vs. Async | Synchronous | Asynchronous |
Querying | No querying support | Supports indexing and complex queries |
Data Storage Format | Strings only | Supports rich data (objects, arrays) |
Browser Support | Excellent | Good, with modern browsers |
When to Use Local Storage vs. IndexedDB
Use Local Storage when:
- You need to store simple key-value pairs.
- Data is relatively small (less than 5MB).
- You don’t need advanced querying or complex data storage.
- The data can be easily represented as a string.
Use IndexedDB when:
- You need to store large amounts of data.
- The data is complex and requires structured storage (objects, arrays, binary data).
- You require querying, indexing, or more advanced data management.
- Your app needs to function offline or store large datasets (e.g., for a PWA or a data-intensive app).
Combining Local Storage and IndexedDB
In many real-world applications, both Local Storage and IndexedDB can be used together. For example, you can store small user preferences or authentication tokens in Local Storage, while using IndexedDB for offline storage or caching large datasets.
// Example: Storing a user preference in Local Storage and user data in IndexedDB
// Save theme preference in Local Storage
localStorage.setItem('theme', 'dark');
// Save large user data in IndexedDB
let dbRequest = indexedDB.open('userDB', 1);
dbRequest.onsuccess = function(event) {
let db = event.target.result;
let transaction = db.transaction(['users'], 'readwrite');
let store = transaction.objectStore('users');
store.add({ id: 2, name: 'Jane Smith', email: 'jane@example.com' });
};
Conclusion
Both Local Storage and IndexedDB have their place in modern web development. Local Storage is simple, fast, and perfect for small data, while IndexedDB provides a more robust solution for handling complex, large-scale client-side storage needs. By understanding the strengths and use cases of both, you can choose the right tool for the job and build more efficient, scalable web applications. Whether you're working on a simple website or a sophisticated PWA, mastering client-side data management is key to optimizing user experience.
FAQs
1. Can I store objects in Local Storage?
No, Local Storage only supports storing strings. However, you can serialize objects to strings using `JSON.stringify()` and deserialize them using `JSON.parse()`.
2. Is IndexedDB supported in all browsers?
IndexedDB is supported by most modern browsers, including Chrome, Firefox, Edge, and Safari. However, it's always a good idea to check compatibility for older browsers or mobile versions.
3. Can I use IndexedDB to store files?
Yes, IndexedDB allows you to store binary data, including files and blobs. This is useful for applications that need to handle media files or large amounts of data.
4. Is Local Storage secure?
Local Storage is not secure and should not be used for sensitive information like passwords. Data stored in Local Storage can be easily accessed by anyone with access to the browser’s developer tools.
5. Can I use both Local Storage and IndexedDB in the same application?
Yes, you can use both Local Storage and IndexedDB in the same application for different purposes, depending on the complexity of the data and the storage needs.